tak jsem měl čas a podíval se na to.. sice nedělám v Javě vůbec ale takto teda moje "garáž" vypadá:
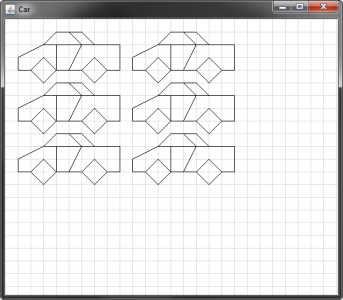
Dělal jsem to tím jak jsem říkal.. prostě třída Auto, kde si vytvoříš jedno auto a potom pomocí offsetů jen posuneš podle pozice X a Y na jinou pozici celý auto
při vykreslení, jen upravuješ body o určitou pozici
Objekt Pera (strará se o čáry)
public class Pen {
public static final int STEP = 20;
private List<Line> lines = new ArrayList<>();
private Point lastPos = new Point();
public void newLine(int x1, int y1, int x2, int y2) {
Line l = new Line(x1 * STEP, y1 * STEP, x2 * STEP, y2 * STEP);
lines.add(l);
lastPos = l.getEnd();
}
public void line(int x, int y) {
Line l = new Line(lastPos.x, lastPos.y, x * STEP, y * STEP);
lines.add(l);
lastPos = l.getEnd();
}
public void draw(Graphics g, int offsetX, int offsetY) {
for (Line line : lines) {
line.draw(g, offsetX * STEP, offsetY * STEP);
}
}
}
Objekt jedné čáry
public class Line {
private Point begin;
private Point end;
public Line(int x1, int y1, int x2, int y2) {
begin = new Point(x1, y1);
end = new Point(x2, y2);
}
public Point getEnd() {
return end;
}
public void draw(Graphics g, int offsetX, int offsetY) {
g.drawLine(begin.x + offsetX, begin.y + offsetY, end.x + offsetX, end.y + offsetY);
}
}
a objekt auta
public class Car {
private Pen pen = new Pen();
private Point offset = new Point();
public Car(int x, int y) {
offset = new Point(x, y);
// základní karoserie
pen.newLine(1, 3, 0, 3);
pen.line(0, 2);
pen.line(2, 1);
pen.line(8, 1);
pen.line(8, 3);
pen.line(7, 3);
// pravé kolo
pen.newLine(7, 3, 6, 4);
pen.line(5, 3);
pen.line(6, 2);
pen.line(7, 3);
// levé kolo
pen.newLine(1, 3, 2, 2);
pen.line(3, 3);
pen.line(2, 4);
pen.line(1, 3);
// spojení mezi koly + čára na předním kole nahoru
pen.newLine(5, 3, 3, 3);
pen.line(3, 1);
// čára ve středu karoserie a přes sklo
pen.newLine(4, 3, 5, 1);
pen.line(4, 0);
// střecha
pen.newLine(6, 1, 5, 0);
pen.line(3, 0);
pen.line(2, 1);
}
public void draw(Graphics g) {
pen.draw(g, offset.x, offset.y);
}
}
V mainu pak můžeš mí třeba toto:
public class MainWindow extends JFrame {
private List<Car> cars = new ArrayList<>();
public MainWindow() {
initComponents();
createBufferStrategy(2);
cars.add(new Car(1, 1));
cars.add(new Car(10, 1));
cars.add(new Car(1, 5));
cars.add(new Car(10, 5));
cars.add(new Car(1, 9));
cars.add(new Car(10, 9));
}
private void drawGrid(Graphics g) {
final int size = (gPanel.getWidth() > gPanel.getHeight()) ? gPanel.getWidth() : gPanel.getHeight();
int n = Pen.STEP;
for (int i = 0; i < size; i += Pen.STEP) {
g.drawLine(0, n, gPanel.getWidth(), n);
g.drawLine(n, 0, n, gPanel.getHeight());
n += Pen.STEP;
}
}
@Override
public void paint(Graphics g) {
super.paint(g);
Graphics gp = gPanel.getGraphics();
gp.setColor(new Color(220, 220, 220));
drawGrid(gp);
gp.setColor(Color.black);
for (Car car : cars) {
car.draw(gp);
}
}
// Generated code...
}
Kdyby bylo něco nejasný, tak comment... nemam to dělaný zrovna nejlepším řešením, ale pro ty učely více aut to funguje =D